How to Read From a Text File in C++ No Fstream
What is file handling in C++?
Files store information permanently in a storage device. With file handling, the output from a plan can exist stored in a file. Various operations tin can be performed on the data while in the file.
A stream is an abstraction of a device where input/output operations are performed. Yous tin can stand for a stream as either a destination or a source of characters of indefinite length. This will be determined by their usage. C++ provides you with a library that comes with methods for file handling. Allow us discuss it.
In this c++ tutorial, you will larn:
- What is file handling in C++?
- The fstream Library
- How to Open up Files
- How to Close Files
- How to Write to Files
- How to Read from Files
The fstream Library
The fstream library provides C++ programmers with three classes for working with files. These classes include:
- ofstream– This class represents an output stream. It's used for creating files and writing information to files.
- ifstream– This grade represents an input stream. Information technology's used for reading information from information files.
- fstream– This class mostly represents a file stream. It comes with ofstream/ifstream capabilities. This means information technology's capable of creating files, writing to files, reading from information files.
The following image makes it simple to understand:
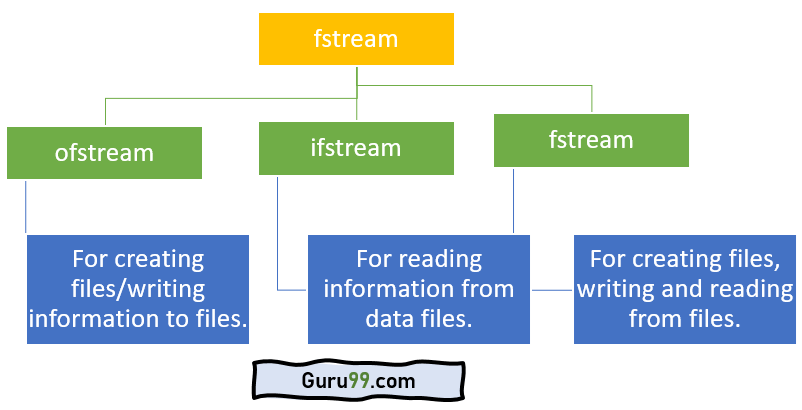
To utilize the in a higher place classes of the fstream library, you must include it in your program as a header file. Of course, you will use the #include preprocessor directive. You lot must also include the iostream header file.
How to Open Files
Before performing any performance on a file, y'all must first open it. If yous need to write to the file, open up it using fstream or ofstream objects. If you but need to read from the file, open up it using the ifstream object.
The iii objects, that is, fstream, ofstream, and ifstream, have the open() office divers in them. The function takes this syntax:
open (file_name, mode);
- The file_name parameter denotes the name of the file to open.
- The mode parameter is optional. It tin take any of the following values:
Value | Description |
---|---|
ios:: app | The Suspend mode. The output sent to the file is appended to information technology. |
ios::ate | It opens the file for the output then moves the read and write control to file's end. |
ios::in | It opens the file for a read. |
ios::out | It opens the file for a write. |
ios::trunc | If a file exists, the file elements should be truncated prior to its opening. |
Information technology is possible to employ 2 modes at the aforementioned time. You combine them using the | (OR) operator.
Instance 1:
#include <iostream> #include <fstream> using namespace std; int principal() { fstream my_file; my_file.open("my_file", ios::out); if (!my_file) { cout << "File not created!"; } else { cout << "File created successfully!"; my_file.close(); } return 0; }
Output:
Here is a screenshot of the lawmaking:
Lawmaking Explanation:
- Include the iostream header file in the program to use its functions.
- Include the fstream header file in the programme to use its classes.
- Include the std namespace in our code to use its classes without calling it.
- Call the main() function. The program logic should go within its trunk.
- Create an object of the fstream class and requite it the name my_file.
- Utilize the open() function on the above object to create a new file. The out mode allows u.s.a. to write into the file.
- Use if statement to check whether file creation failed.
- Message to print on the panel if the file was not created.
- Terminate of the torso of if statement.
- Use an else statement to state what to do if the file was created.
- Message to impress on the panel if the file was created.
- Utilize the close() function on the object to close the file.
- Finish of the body of the else statement.
- The plan must return value if it completes successfully.
- Terminate of the chief() function torso.
How to Close Files
One time a C++ program terminates, it automatically
- flushes the streams
- releases the allocated memory
- closes opened files.
However, as a developer, you lot should learn to close open files before the program terminates.
The fstream, ofstream, and ifstream objects have the close() part for closing files. The function takes this syntax:
void shut();
How to Write to Files
Y'all can write to file right from your C++ program. Y'all use stream insertion operator (<<) for this. The text to exist written to the file should be enclosed within double-quotes.
Let united states of america demonstrate this.
Example 2:
#include <iostream> #include <fstream> using namespace std; int main() { fstream my_file; my_file.open("my_file.txt", ios::out); if (!my_file) { cout << "File non created!"; } else { cout << "File created successfully!"; my_file << "Guru99"; my_file.shut(); } render 0; }
Output:
Here is a screenshot of the lawmaking:
Code Explanation:
- Include the iostream header file in the programme to use its functions.
- Include the fstream header file in the plan to use its classes.
- Include the std namespace in the program to use its classes without calling it.
- Call the main() part. The programme logic should be added within the body of this office.
- Create an case of the fstream grade and give it the proper name my_file.
- Use the open() function to create a new file named my_file.txt. The file will exist opened in the out manner for writing into it.
- Use an if statement to check whether the file has not been opened.
- Text to print on the console if the file is non opened.
- End of the body of the if statement.
- Utilise an else statement to state what to practice if the file was created.
- Text to print on the console if the file was created.
- Write some text to the created file.
- Employ the close() function to shut the file.
- End of the body of the else statement.
- The program must return value upon successful completion.
- Stop of the body of the primary() part.
How to Read from Files
Y'all can read data from files into your C++ program. This is possible using stream extraction operator (>>). You utilise the operator in the same fashion y'all use it to read user input from the keyboard. However, instead of using the cin object, y'all employ the ifstream/ fstream object.
Example 3:
#include <iostream> #include <fstream> using namespace std; int main() { fstream my_file; my_file.open("my_file.txt", ios::in); if (!my_file) { cout << "No such file"; } else { char ch; while (i) { my_file >> ch; if (my_file.eof()) break; cout << ch; } } my_file.close(); render 0; }
Output:
No such file
Hither is a screenshot of the code:
Code Explanation:
- Include the iostream header file in the programme to utilise its functions.
- Include the fstream header file in the programme to employ its classes.
- Include the std namespace in the programme to use its classes without calling it.
- Call the main() function. The plan logic should exist added within the body of this function.
- Create an instance of the fstream class and give it the proper name my_file.
- Use the open() function to create a new file named my_file.txt. The file volition exist opened in the in mode for reading from it.
- Use an if statement to check whether the file does not exist.
- Text to print on the console if the file is not found.
- Cease of the body of the if argument.
- Use an else statement to land what to exercise if the file is institute.
- Create a char variable named ch.
- Create a while loop for iterating over the file contents.
- Write/shop contents of the file in the variable ch.
- Use an if condition and eof() function that is, end of the file, to ensure the compiler keeps on reading from the file if the stop is not reached.
- Employ a intermission argument to cease reading from the file once the end is reached.
- Print the contents of variable ch on the console.
- Terminate of the while body.
- End of the trunk of the else statement.
- Call the close() function to close the file.
- The program must return value upon successful completion.
- Stop of the torso of the main() function.
Summary:
- With file treatment, the output of a plan tin can be sent and stored in a file.
- A number of operations tin can then be practical to the data while in the file.
- A stream is an abstraction that represents a device where input/output operations are performed.
- A stream tin can exist represented every bit either destination or source of characters of indefinite length.
- The fstream library provides C++ programmers with methods for file treatment.
- To use the library, you must include it in your program using the #include preprocessor directive.
Source: https://www.guru99.com/cpp-file-read-write-open.html
0 Response to "How to Read From a Text File in C++ No Fstream"
Post a Comment